*DISCLAIMER: THIS ARTICLE IS NOT A SCALA TUTORIAL, IT'S A BRIEF INTRODUCTION TO SCALA LANGUAGE AND THE REASONS WHY YOU SHOULD BE INTERESTED IN LEARNING IT.
In my last blog post I wrote about alternative languages to use in the JVM and the reasons why it's good to have more than one in your toolbox. Continuing with this idea we are going to analyze in more detail one of the most solid alternative JVM languages we have at the moment: Scala. I had to use Scala for a big, real time social media data analysis system I worked on last year and while at first it was hard, I ended up having a blast using it.
Why Scala?
According to several sources [1, 2] Scala is the second most popular JVM language after Java itself, so that makes it an obvious candidate if you are looking for an alternative to Java but you want to stay using the JVM. With a mature toolset, a strong community and technical support, it’s widely used and well understood.
Where is Scala used?
There are a few well known open-source projects using Scala: Apache Spark cluster computing solution and Apache Kafka a publisher subscriber message queue, to name two of them. Twitter jumped from Ruby to Scala in 2009 for performance reasons and have since contributed a lot to the language development and adoption. Scala has been widely adopted across multiple areas by companies such as Netflix, Twitter, Facebook and Amazon and is commonly used for entire applications, most notably Big Data. It’s safe to say the Big Data feature set is the "Killer Domain" of Scala.
What is Scala?
Scala is short for “Scalable Language” and it is a general purpose programming language. It's designed to embrace multiple programming paradigms, mainly merging object oriented and functional programming within a strong static type system. Scala was also designed to tackle some of the most important shortcomings and criticisms of Java.
Scala is more expressive, concise, and safer than Java. It has a strong emphasis on immutability, lazy evaluation, solid functional programming concepts and improved object oriented constructs, including a safer static type system.
Scala was also designed to have an easy start path to using it effectively if you are coming from Java, C# or a similar language, and so it can be used as a "better Java than Java" with a slightly different syntax, but Scala also incorporates more advanced functional and type constructs than Java, making Scala a completely different language capable of expressing problems in different ways.
Why Scala over Java 8?
Java 8 introduced some important functional programming capabilities, but how do these compare the ones in Scala? I won’t make a real comparison here because that would require a separate post, but I will say that Java 8 functional programming capabilities are a valuable addition to the language but are not as powerful as Scala’s capabilities. Scala also has an expensive and flexible syntax with a strong domain of specific language building capabilities.
Scala and functional programming
Scala not only supports but extends all of the object-oriented features available in Java, and its greatest strength resides in its functional programming capabilities. This allows Scala programs to be written in an almost completely functional style, an almost completely object-oriented style, or the idiomatic Scala way that is a mix of both paradigms. Some of the advanced functional programming capabilities included in Scala are:
- No distinction between statements and expressions
- Type inference
- Anonymous functions with capturing semantics (i.e., closures)
- Immutable variables and objects
- Lazy evaluation
- Delimited continuations
- Higher-order functions
- Nested functions
- Currying
- Pattern matching
- Algebraic data types
- Tuples
Scala, Data Immutability and concurrency
One of the biggest problems in concurrency programming is mutable state. Mutable state requires a lot of effort to ensure correctness, it’s very prone to error and it produces bugs difficult to find and fix. It also makes code that is hard to understand, analyze, predict and reason about. Functional programming solves this problem by not having mutable state so no function can have side effects.Though Scala promotes this way of thinking, it doesn't force it. You can still have mutable state in Scala, it's just strongly discouraged. The Scala mindset is to use mutable state only if you have a very good reason to do it.
How does Scala look like compared to Java
Methods
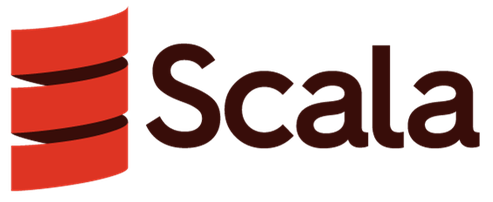
Scala:
Java:
In Scala, types are declared in a postfix way but are only needed in places where the compiler can't infer them. Method definitions start with the reserved word def, code block braces are not required for single statement code statements, and the return operator is optional because methods return the value of their last statement. Casts in Scala are calls to asInstanceOf method, and Scala constants are declared with val, with variables been declared with var.
Classes
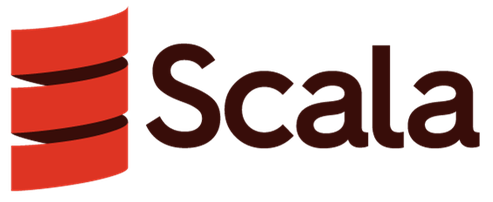
Scala:
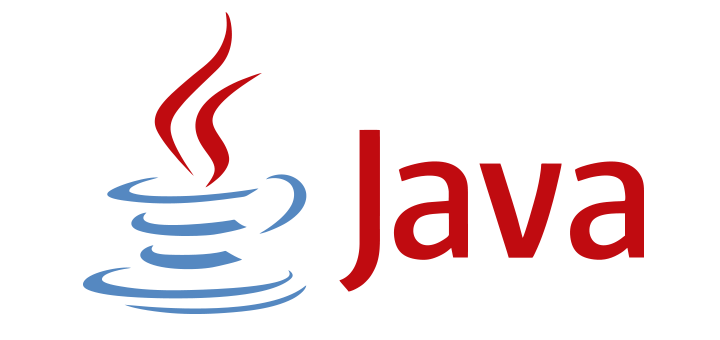
Java:
As you can see, there is a lot less syntactic boilerplate in Scala than there is in Java. That concept of syntactic simplicity and flexibility is extended all over the language making it possible to easily define Domain Specific Languages in Scala without the need of language extensions. Another important difference between Java and Scale is that Scala has a unified type system without the Java distinction between primitive types and reference types.
The dark side of Scala
Scala is not perfect, no programming language is. Some outstanding issues include:
- Slow compilation in comparison to Java. This has been improved over time with each new compiler version and it will continue to improve, but the Scala compiler has a lot more work to do compared to Java so it will never be as fast as Java.
- Binary incompatibilities between compiler versions. In the past this issue was very bad, including binary incompatibilities even between some patch versions. But, from 2.10.0 on, Scala patch versions have become binary compatible making this issue a lot better, but still far from perfect. This is especially relevant for library developers that need to support multiple Scala versions of their projects.
- Scala complexity. Scala is complex with a steep learning curve and is not as popular as other languages. This makes growing a Scala development team harder than a Java teame. The good thing is you can start using Scala as a object-oriented language that’s not far off from Java and incorporates more advanced techniques over time. This is even better now that Java 8 is in extend usage with some functional programming concepts already backed in.
Do you want to learn more about Scala?
So you’re interested in learning about this OO/Functional hybrid language?
My first advice is do it a step at a time, like Toby Weston suggested in this blog. You can start using Scala from an OO mindset and introduce the Functional aspects slowly until you and your team feel comfortable.
Some Scala learning resources
- The Scala Programming Language website tutorials: http://www.scala-lang.org/tutorials
- Functional Programming Principles in Scala by Martin Odersky: https://www.coursera.org/learn/progfun1
- https://www.scala-exercises.org/
- http://allaboutscala.com/