Have you ever wondered how your favorite text editor works under the hood? How does it read your files and has access to save them? How does it remember your personal settings, workspaces and manages to work across multiple OS's?
We really don't give much thought to this, we just use it as long as it helps us accomplish our tasks efficiently.
Node's file system API helps us achieve all this and more
VSCode runs on Electron and because of that it is of no surprise that they use Node to access the file system. But how does this API work?
Node's API tackles the CRUD operations both for files and directories. It is also worth mentioning that Node's API offers synchronous and asynchronous methods to execute each operation. We will be focusing on the asynchronous methods for the purpose of this article.
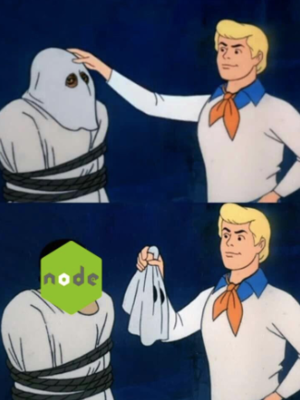
READ
Reading files is one of the simplest actions to take as you do not touch or modify any file content, but simply consume its existing information.
To do this, Node gives us the `readFile` method, part of its fs package. The following snippet demonstrate its usage:

This method retrieves the data of the given file and stores it in memory before actually returning the data to you. The downside of this is that the more file content we have, the more RAM has to be put in use, so it is recommended to read in chunks or streams, to batch the content put on RAM.

Once you have the data, it's returned to you in Buffer and, although you can parse it to your convenience, you can pass down an optional parameter to the function and have it return the value in a requested format.
CREATE/UPDATE
To create a simple file we must give our function a file descriptor (name and path), some data to write in it and the callback function. There is an “additional options” object which can include mode properties, a string specifying its encoding or these series of flags:
- r+ open the file for reading and writing.
- w+ open the file for reading and writing, positioning the stream at the beginning of the file. The file is created if not existing.
- a open the file for writing, positioning the stream at the end of the file. The file is created if not existing.
- a+ open the file for reading and writing, positioning the stream at the end of the file. The file is created if not existing.

It is worth mentioning that fs.writeFile() completely wipes out the previous content of the file. If interested in adding/appending content you can use fs.appendFile() as an alternative.
DELETE
If interested in removing a file rather than adding content to one or creating a new one, you use `fs.unlink`.
This method removes a file or a symlink. It expects the file descriptor and the callback function. This function receives nothing but an error argument in case the operation fails.

Directories
READ
When reading what’s inside of a directory, Node gives us the `readdir` method.
Let’s take a look at how it looks in code:

This method’s arguments are: directory path, options object, and the callback function. This callback, takes in two params, one to capture the error if anything goes wrong in runtime, and the entries, which is an array of the elements held inside the directory.
WRITE
The writing method is a bit different, not in how it’s called, or the arguments received, but definitely in how it behaves, as this time you cannot append content to the directories.
Below is an example of the `mkdir` method:

It is called with two parameters, one being the name of the new directory and the other one the callback function which holds only one argument. The callback function is the error in case the method fails to create the new directory. However, just as the previous method, this one receives an options object like the second parameter but it is optional.
DELETE
Finally, we have `rmdir`. This method receives just two arguments: the path of the directory to be deleted (this method does not work recursively so the directory must be empty when called) and the callback function, to handle errors if needed.

If you want to dig in deeper about this topic make sure to check out Node's FS API and VSCode's repo.
Node FS API: https://nodejs.org/api/fs.html
VSCode repo: https://github.com/microsoft/vscode